I've decided that I'm going to make a "shooter" type game in which the player will be represented by a gun or cannon which is fixed to the middle of the bottom of the stage. The barrel of the gun will follow the mouse cursor and a bullet of some sort will be fired when the mouse is clicked.
Although this is quite a simple game, I have chosen it because I am confident that I can create the mechanics of this game relatively easily, and I figure it's best to start with a simple project that I have a chance of completing. I can also add things like upgrades, different types of enemies, different types of weapons etc. to make the game more interesting.
Player Creation
![]() |
The "gun" |
So the approach I am going to take to this game is first to create the main game mechanics, and then I can build things like the main menu and inter-level screens later. So first things first, I create a new flash file with actionscript 2.0. I am using AS2 because that is what I have some previous experience with, and from what I've seen AS3 is considerably different to AS2 in terms of structure and syntax. So for the moment I'm just going to stick to what I know. Later, if I'm successful with this project, I might try to convert it to AS3 as a way to learn it.
I have setup the flash movie to be 550 x 800 px and a frame rate of 30 fps. Now the first thing I need to do is create a movieclip which is going to represent the player and place it on the screen. I'm not the most creative person so for the moment I'm going to use pretty simple sprites that I can create with simple shapes in flash. I've created a gun out of a grey circle, square, and rectangle which I've then converted to a symbol (right click, convert to symbol). Be sure to give it a name (I've called mine gunbarrell) and in the linkage box click Export for ActionScript. This will allow you to reference the object from the code.
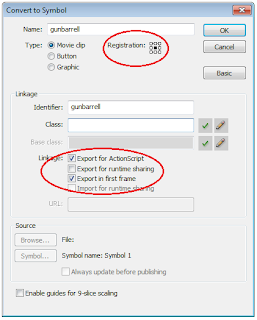
Once you convert it to a symbol it will appear in your library. You can now delete the gun off the stage, because we will be creating an instance dynamically in the code.
Now before we jump into the movement code we need to do one more thing - change the registration of the gunbarrell movieclip. Because I want the gun barrel to rotate around the center of the circle, as opposed to the center of the whole movieclip, we need to manually move the registration point to the center of the circle. To do this double click the gunbarrell movieclip in the library, which will take you to the symbol editing mode. A small black cross represents the registration point so drag this to the center of the circle, then click the blue back arrow on the toolbar to return to the stage.
Coding Player Movement
Okay, so we're ready to start coding! Right click on the first (and only) frame in the timeline and click actions. This is where we will be writing most of the code for the game. The first thing we need to do is attach a copy of gunbarrell to the stage:
_root.attachMovie("gunbarrell","gunbarrell",_root.getNextHighestDepth())
Pretty simple. the function attachMovie(idname, newname, depth) attaches a movie clip to the stage. idname is the name of the object in the library, newname is the name used to refer to the object in the code and depth is, well, the objects depth.
Now that we have the gunbarrell attached to the stage we can change it's x and y values, and it's rotation easily. So let's move it to the center of the bottom of the screen.
gunbarrell._x = 275; gunbarrell._y = 780;
So, what we want is for the gun barrell to rotate in such a way as the end of the barrell always follows the mouse pointer. It took me a little while to figure this out, so what I'm going to do is just post the whole function and then run through it.
onMouseMove = function () { mouse_xdist = _root._xmouse-gunbarrell._x; mouse_ydist = _root._ymouse-gunbarrell._y; // calculate the angle barrell_rad = Math.atan2(mouse_ydist, mouse_xdist); // convert to degrees and set rotation barrell_angle = toDeg(barrell_rad) + 90; gunbarrell._rotation = barrell_angle; } function toDeg(Rad:Number){ return (Rad * 180 / Math.PI); }
Ok, so the first line just creates a new function which will be executed whenever the mouse moves. Then we set 2 variables, mouse_xdist and mouse_ydist to the x and y distances from the mouse pointer to the registration point of gunbarrell. These two distances effectively form the two non-hypotenuse sides of a right angle triangle. We want to find the angle that the barrell is pointing, which is also the bottom angle of this triangle, and we do this in the 5th line using trigonometry.
The reason we use the atan2 function (and why we don't need to worry about negative angles) is explained best by flash-creations.com:
atan2 is often more useful than atan in applications involving rotation by a specified amount because it returns a positive beta for all angles between 0 and 180 degrees (even when x is negative). Thus it eliminates the need for extra code to deal with different values in different quadrants of the circle.
Because the actionscript trig functions return results in radians, but the _rotation attribute of a movieclip is accepted in degrees, we need to convert barrell_rad to degrees so I have written another function, toDeg(Rad), to do this.
The last thing I did today was create the bullet shooting and movement, however I'll post that in the next post because this one is long enough already. Should be up in a few hours!
Thanks,i tried coding games once,too hard for me... lol
ReplyDeleteHow much formal education or classes did you need to learn all of this? Self-taught?
ReplyDelete@kitsmoleculargenetics Thanks for checking out the blog! This is all self taught. I did a basic programming class in highschool about 9 years ago but it was just basic qbasic and visualbasic stuff. I just found I loved it and since then have tinkered with a few different languages. I like flash & actionscript because it makes the graphical side of things way easier, and lets me focus on what I'm good at which is the logic.
ReplyDelete